Unveiling The Magic Of Metaprogramming With Spring Boot Data Visualization
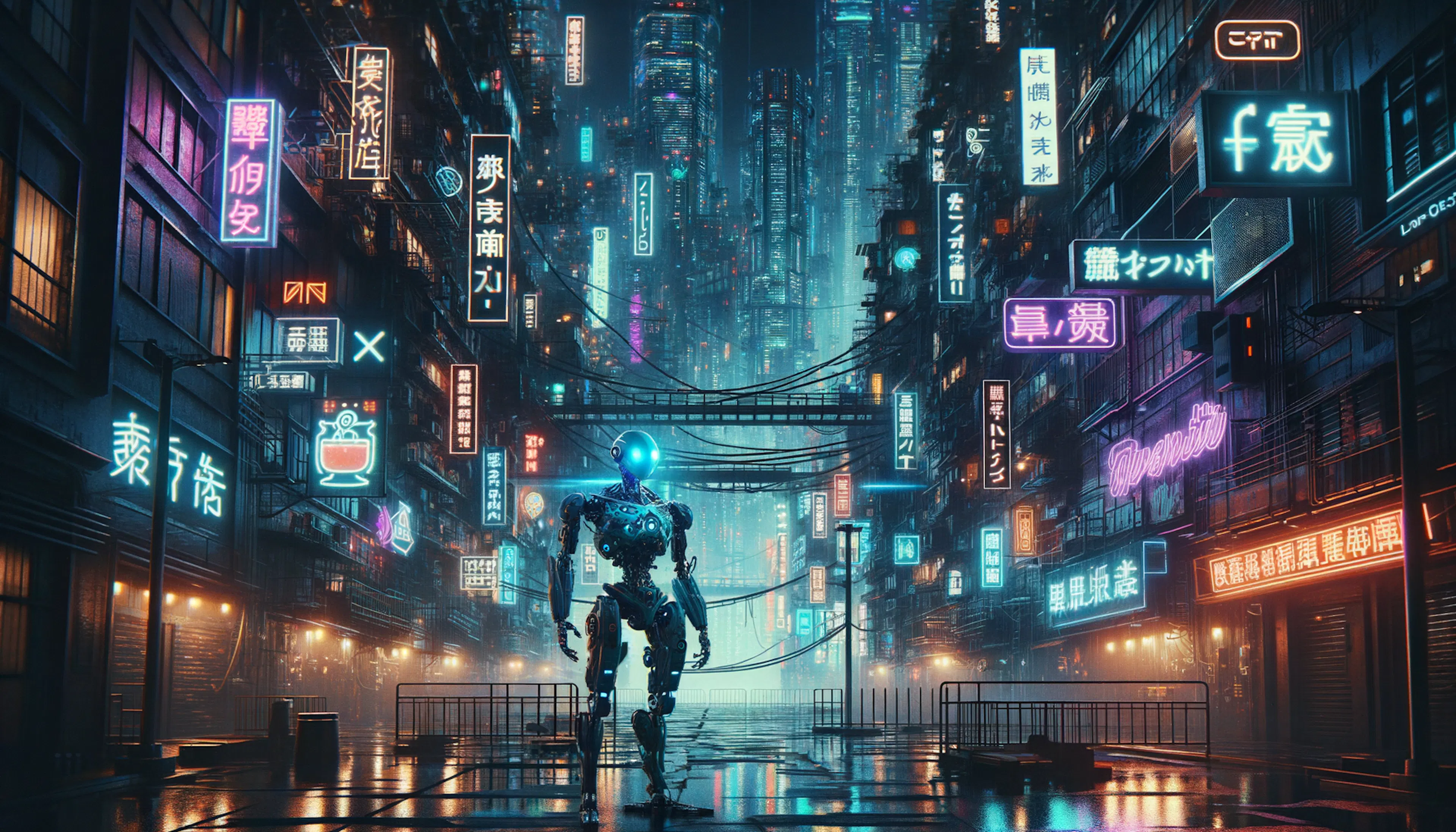
In the ever-evolving landscape of software development, metaprogramming stands out as a powerful technique that allows developers to write code that writes code. This concept, while initially intimidating, opens up a world of possibilities for creating more flexible, efficient, and dynamic applications. Today, we'll explore how to harness the power of metaprogramming in Spring Boot through a data visualization mini-project.
The Essence of Metaprogramming
Metaprogramming is the art of writing code that manipulates, generates, or analyzes other code. In essence, it's programming that treats code as data. This powerful paradigm allows developers to:
- Reduce boilerplate code
- Enhance flexibility in software design
- Create more
dynamic
and adaptable applications
By leveraging metaprogramming techniques, we can build systems that evolve and adapt to changing requirements with minimal manual intervention.
Setting Up Our Spring Boot Project
To demonstrate metaprogramming in action, we'll create a simple data visualization application using Spring Boot. This project will dynamically generate data models and corresponding visualizations based on user input.
- Start by creating a new Spring Boot project using your preferred IDE or the Spring Initializer.
- Add the following dependencies to your
pom.xml
:- Spring Web
- Spring Data JPA
- H2 Database
- Thymeleaf (for our front-end)
Remember, the goal here is not just to create a data visualization tool, but to showcase how metaprogramming can make our application more flexible and powerful.
Implementing Dynamic Model Generation
The heart of our metaprogramming showcase lies in dynamically generating data models based on user input. Here's how we can achieve this:
@RestController
public class ModelGeneratorController {
@PostMapping("/generate-model")
public String generateModel(@RequestBody Map<String, String> fields) {
StringBuilder modelCode = new StringBuilder("public class DynamicModel {\n");
for (Map.Entry<String, String> entry : fields.entrySet()) {
modelCode.append(" private ")
.append(entry.getValue())
.append(" ")
.append(entry.getKey())
.append(";\n");
}
modelCode.append("}");
return modelCode.toString();
}
}
This controller takes a JSON payload describing the fields and their types, then dynamically generates a Java class definition. While this is a simplified example, it demonstrates the core concept of code generation at runtime.
Dynamic Visualization Generation
With our model in place, let's create a system that can dynamically generate visualizations based on the model structure:
@Service
public class VisualizationService {
public String generateVisualization(String modelDefinition) {
// Parse the model definition
// Extract field names and types
// Generate appropriate visualization code (e.g., HTML/JavaScript for charts)
return "<div id='chart'></div><script>// Chart generation code here</script>";
}
}
This service would analyze the dynamically generated model and create an appropriate visualization. The actual implementation would involve parsing the model definition and using a charting library to generate the visualization code.
Bringing It All Together
To complete our mini-project, we'll create a controller that ties everything together:
@Controller
public class DataVisualizationController {
@Autowired
private ModelGeneratorController modelGenerator;
@Autowired
private VisualizationService visualizationService;
@PostMapping("/visualize")
public String visualizeData(@RequestBody Map<String, String> fields, Model model) {
String generatedModel = modelGenerator.generateModel(fields);
String visualization = visualizationService.generateVisualization(generatedModel);
model.addAttribute("visualization", visualization);
return "visualization";
}
}
This controller orchestrates the process:
- It receives the field definitions from the user
- Generates a dynamic model
- Creates a visualization based on that model
- Renders the result using a Thymeleaf template
Reflecting on the Power of Metaprogramming
By leveraging metaprogramming in our Spring Boot application, we've created a system that can adapt to various data structures without requiring code changes. This flexibility has profound implications:
- Rapid prototyping: Developers can quickly create and visualize new data models without writing extensive code.
- Customization: End-users can define their own data structures and visualizations, empowering them to explore data in ways that suit their specific needs.
Maintenance efficiency
: As new requirements emerge, the system can adapt without extensive refactoring.
The intersection of metaprogramming and modern frameworks like Spring Boot opens up exciting possibilities for creating more adaptive and user-centric applications. As we continue to push the boundaries of what's possible in software development, techniques like these will play an increasingly crucial role in shaping the future of our digital landscape.
As we conclude, it's worth pondering: How might the widespread adoption of metaprogramming techniques reshape the role of developers and the way we approach software design in the coming years?