Unlocking The Power Of Metaprogramming In Angular: A Command Line Adventure
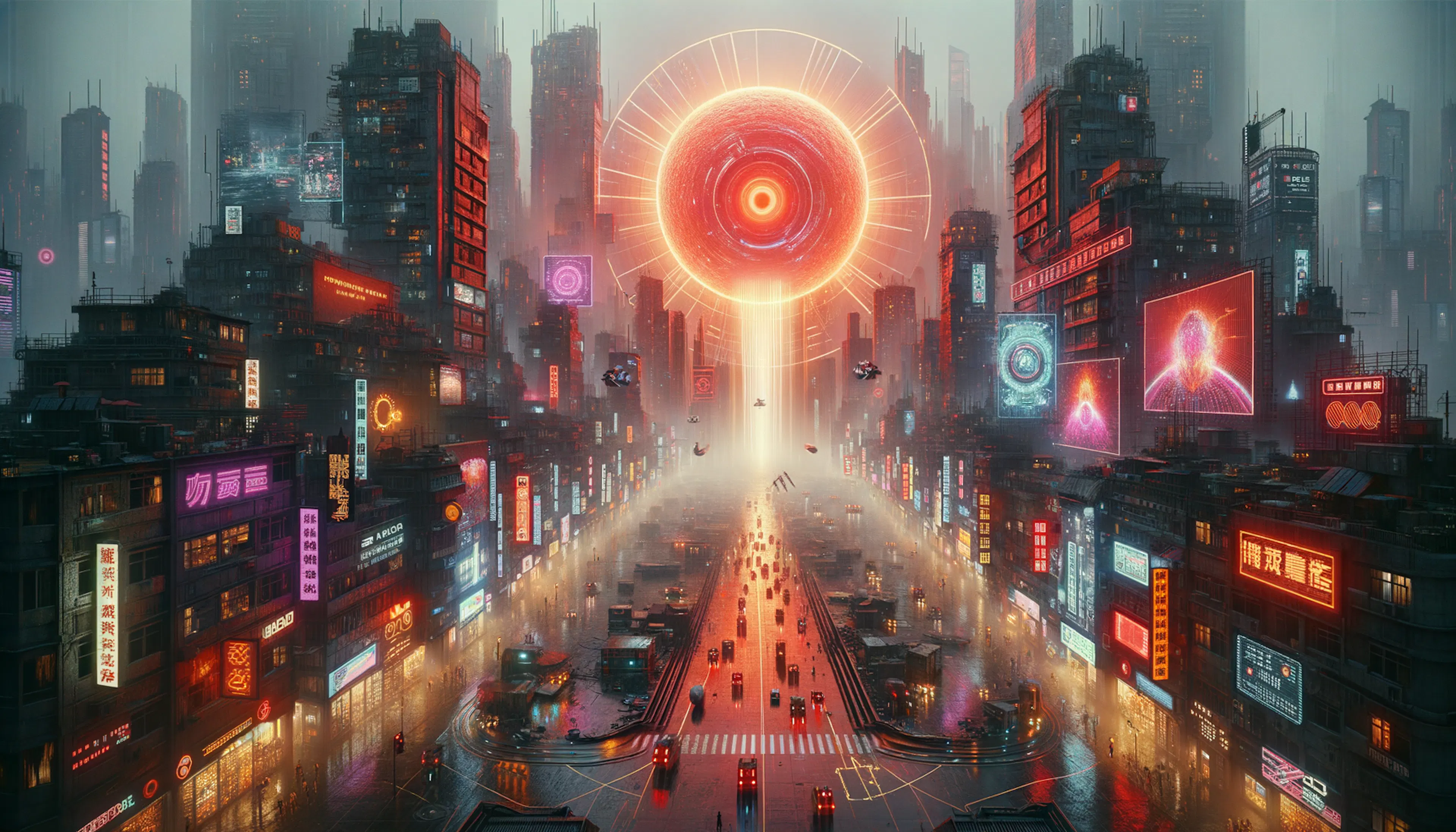
In the ever-evolving landscape of web development, Angular continues to push boundaries and offer developers powerful tools for creating robust applications. Today, we're diving into the fascinating world of metaprogramming and exploring how it can be leveraged within Angular through a command-line application. Get ready to elevate your coding skills and unlock new possibilities!
Understanding Metaprogramming in Angular
Metaprogramming, at its core, is the practice of writing code that can manipulate or generate other code. In the context of Angular, this concept opens up exciting avenues for creating more dynamic and flexible applications. Let's explore how we can harness this power through a mini-project.
Setting Up the Project
To begin our metaprogramming journey, we'll need to set up a new Angular project. Follow these steps:
- Open your terminal and navigate to your desired directory
- Run
ng new angular-metaprogramming-cli
- Choose your preferred options for the project setup
cd
into the newly created project folder
With our project scaffolded, we're ready to dive into the heart of our metaprogramming adventure.
Creating a Dynamic Component Generator
Our first foray into metaprogramming will involve creating a command-line tool that generates Angular components on the fly. This tool will demonstrate how we can use code to create more code, a fundamental concept in metaprogramming.
Implementing the Generator
- Create a new file called
component-generator.ts
in thesrc
folder - Add the following code:
import * as fs from 'fs';
import * as path from 'path';
export function generateComponent(name: string) {
const templateContent = `
import { Component } from '@angular/core';
@Component({
selector: 'app-${name}',
template: '<p>${name} works!</p>',
styleUrls: ['./${name}.component.css']
})
export class ${capitalizeFirstLetter(name)}Component {
// Component logic here
}
`;
const cssContent = `
/* ${name} component styles */
`;
const componentDir = path.join('src', 'app', name);
fs.mkdirSync(componentDir, { recursive: true });
fs.writeFileSync(path.join(componentDir, `${name}.component.ts`), templateContent);
fs.writeFileSync(path.join(componentDir, `${name}.component.css`), cssContent);
console.log(`Component ${name} generated successfully!`);
}
function capitalizeFirstLetter(string: string) {
return string.charAt(0).toUpperCase() + string.slice(1);
}
This code defines a function that generates a basic Angular component structure based on a provided name.
Integrating with the Command Line
Now that we have our component generator, let's create a command-line interface to interact with it.
-
Install the necessary dependencies:
npm install commander
-
Create a new file called
cli.ts
in the project root:
#!/usr/bin/env node
import { Command } from 'commander';
import { generateComponent } from './src/component-generator';
const program = new Command();
program
.version('1.0.0')
.description('Angular Metaprogramming CLI')
.command('generate-component <name>')
.action((name) => {
generateComponent(name);
});
program.parse(process.argv);
- Update your
package.json
to include the CLI:
{
"scripts": {
"start": "ng serve",
"build": "ng build",
"cli": "ts-node cli.ts"
}
}
Now you can run your CLI tool using:
npm run cli generate-component my-new-component
This command will create a new component named my-new-component
in your Angular project.
Extending the Metaprogramming Capabilities
With our basic component generator in place, we can explore more advanced metaprogramming techniques:
- Dynamic Module Generation: Create a function that generates entire Angular modules based on user input.
- Code Analysis: Implement a tool that analyzes your Angular codebase and provides insights or suggestions for improvements.
- Custom Decorators: Develop custom decorators that add functionality to your components or services at runtime.
"Metaprogramming is not just about writing code that writes code; it's about creating tools that enhance our development process and make our applications more flexible and powerful." - Anonymous Developer
Conclusion: The Future of Angular Development
As we've seen, metaprogramming in Angular opens up a world of possibilities for creating more dynamic, efficient, and maintainable applications. By leveraging these techniques, developers can automate repetitive tasks, create more flexible architectures, and ultimately build better software.
The mini-project we've explored today is just the tip of the iceberg. As you continue to delve into metaprogramming, you'll discover countless ways to enhance your Angular development workflow and create more sophisticated applications.
Food for Thought
As we conclude our exploration of metaprogramming in Angular, consider this question: How might the widespread adoption of metaprogramming techniques change the landscape of web development, and what new challenges or opportunities might arise as a result?
By embracing metaprogramming, we're not just writing code; we're crafting tools that shape the very fabric of our development process. The journey has only just begun – what will you build next?