Revolutionizing Web Development With Protocol Oriented Programming In Django
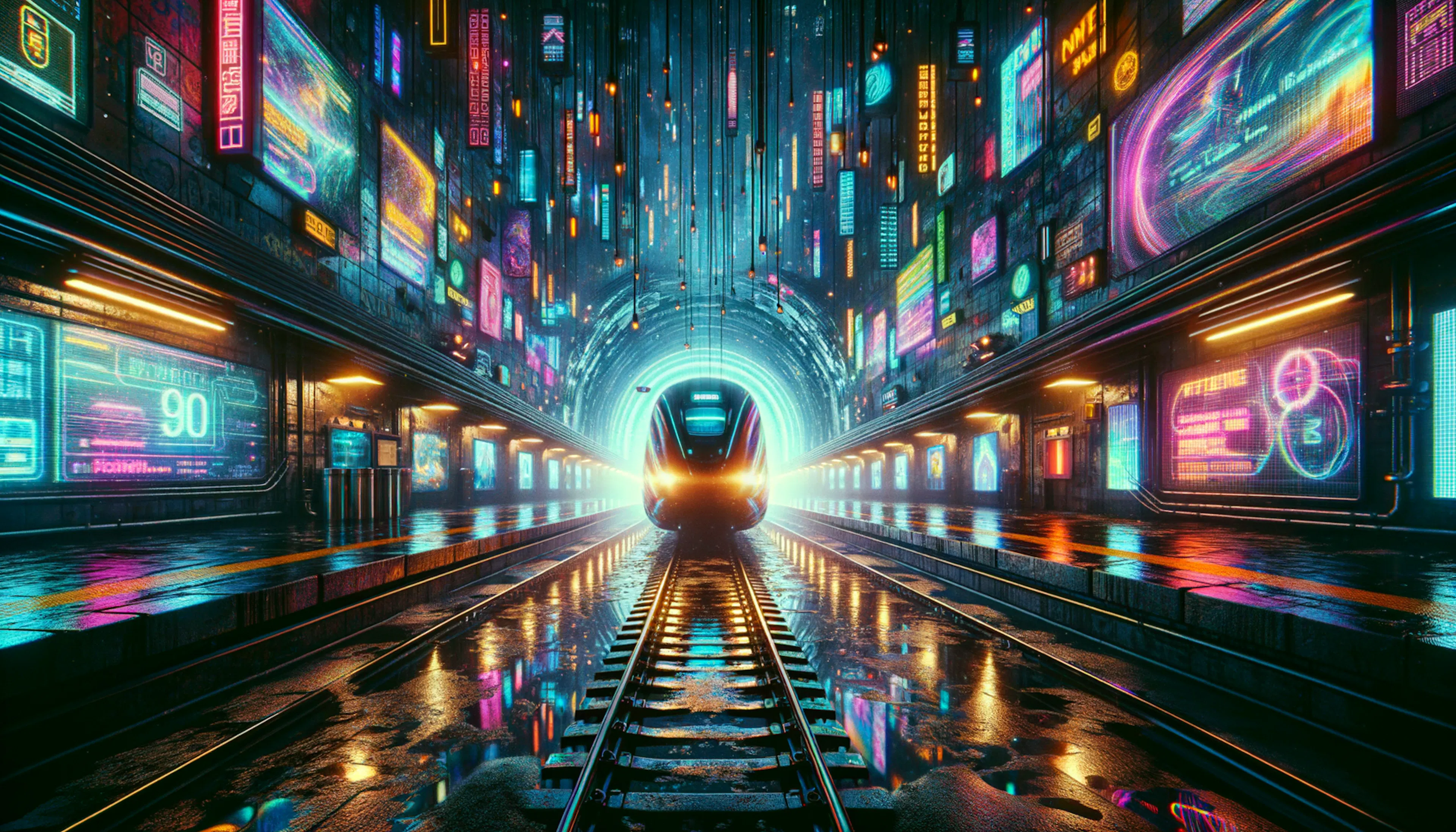
In the ever-evolving landscape of web development, staying ahead of the curve is crucial. Today, we're diving into an exciting concept that's gaining traction: protocol-oriented programming (POP). We'll explore how to implement this paradigm in Django, creating a mini-project that showcases its power and flexibility.
Understanding Protocol-Oriented Programming
Protocol-oriented programming is a design approach that focuses on defining protocols (interfaces) that describe the capabilities of types. This paradigm emphasizes composition over inheritance, leading to more modular and flexible code.
In the context of Django, POP can be implemented using abstract base classes and mixins. These tools allow us to define behavior that can be shared across multiple classes without the rigid hierarchy of traditional inheritance.
Setting Up Our Django Mini-Project
Let's create a simple task management system to demonstrate POP principles. Our project will consist of different types of tasks, each with unique behaviors, but sharing common interfaces.
- First, create a new Django project and app:
django-admin startproject pop_demo
cd pop_demo
python manage.py startapp tasks
- In
tasks/models.py
, let's define our protocols (abstract base classes):
from django.db import models
from abc import ABC, abstractmethod
class TaskProtocol(ABC):
@abstractmethod
def complete(self):
pass
@abstractmethod
def get_priority(self):
pass
class Task(models.Model, TaskProtocol):
title = models.CharField(max_length=200)
completed = models.BooleanField(default=False)
class Meta:
abstract = True
def complete(self):
self.completed = True
self.save()
@abstractmethod
def get_priority(self):
pass
Implementing Concrete Task Types
Now that we have our protocols in place, let's create some concrete task types:
class SimpleTask(Task):
def get_priority(self):
return "Low"
class UrgentTask(Task):
deadline = models.DateTimeField()
def get_priority(self):
return "High"
class RecurringTask(Task):
frequency = models.CharField(max_length=50)
def get_priority(self):
return "Medium"
Each task type implements the get_priority
method differently, showcasing the flexibility of our protocol-based approach.
Creating Views and Templates
To bring our mini-project to life, let's create some views and templates:
- In
tasks/views.py
:
from django.views.generic import ListView
from .models import SimpleTask, UrgentTask, RecurringTask
class TaskListView(ListView):
template_name = 'tasks/task_list.html'
def get_queryset(self):
return list(SimpleTask.objects.all()) + \
list(UrgentTask.objects.all()) + \
list(RecurringTask.objects.all())
- Create a template at
tasks/templates/tasks/task_list.html
:
<h1>Task List</h1>
<ul>
{% for task in object_list %}
<li>
{{ task.title }} - Priority: {{ task.get_priority }}
{% if not task.completed %}
<form method="post" action="{% url 'complete_task' task.id %}">
{% csrf_token %}
<button type="submit">Complete</button>
</form>
{% endif %}
</li>
{% endfor %}
</ul>
Reflecting on the Impact
By implementing protocol-oriented programming in our Django project, we've created a flexible and extensible system for managing different types of tasks. This approach allows us to:
- Easily add new task types without modifying existing code
- Ensure consistency across different implementations
- Improve code reusability through shared protocols
The implications of this paradigm extend beyond our simple example. In larger, more complex systems, POP can lead to:
- Reduced coupling between components
- Increased maintainability of codebases
- Enhanced scalability for growing applications
As we continue to push the boundaries of web development in the 21st century, embracing paradigms like protocol-oriented programming becomes increasingly important. It allows us to create more robust, flexible, and future-proof applications.
"The measure of intelligence is the ability to change." - Albert Einstein
This quote reminds us of the importance of adapting our programming approaches to meet the evolving needs of modern software development.
As we conclude, let's ponder: How might protocol-oriented programming reshape the way we approach complex web applications, and what other traditional programming paradigms could benefit from a fresh perspective?